A folding pipeline ADC generates a serial gray code bit stream starting with MSB and ending with LSB.
A digital circuit converting a 16 bit serial bit stream should be designed.
In Interface electronics a circuit FoldingPipelineTest.asc uses Shift8Gray.asc for conversion.
In this laboratory a digital circuit converting a 16 bit serial bit stream should be designed.
Schematic created with Vivado: 'RTL Analysis', 'Schematic'.
Xilinx Webpack ISE 14.7 running in a virtual machine was used with Spartan 6 device for converting behavioral VHDL to structural VHDL.
Xilinx Vivado was used for simulation. Unfortunately AnalyzeJS.html supports not the Spartan 7 output.
Xilinx Webpack 14.7: Synthesize - XST - Generate Post-Synthesis Simulation Model.
Vivado: After synthesis run Tcl console command: 'write_vhdl <filename>' to get a structural post synthesis description for AnalyzeJS.html.
In the Vivado simulation 3 correct 16-bit output values d[15:0] can be seen.
After doing 'Synthesis - Open synthesized Desing' a synthesized vhd file is generated.
In Vivado a structural VHDL was the generated using TCL command window: write_vhdl <filename>.
.src/sources__1/imports.
Unfortunately AnalyzeJS.html does not support Vivado Spartan 7 devices yet.
In Xilinx Wepack a structural VHDL was the generated:
SGrayBinary_synthesis.vhd
This file was inserted into AnalyzeJS.html.
After pressing 'Process VHDL for Electric (Type: BIT)' an Electric compatible VHDL is generated.
Start Electric and load the librarys sclib.jelib.
A new library (File - New library) and cell (Cell - New Cell) view: 'VHDL' and name: 'SGrayBinary' is created.
The 'SGrayBinary' entity and architecture from AnalyzeJS.html are inserted.
The ':="X"' for c3, c2 and gd in the entity part is removed.
The last semicolon after d__15 is removed.
The components are inserted into the architecture:
architecture Structure of SGrayBinary is
component BUFGP port ( I: in BIT;O: out BIT);
end component;
component FDC port ( C, CLR,D: in BIT;Q: out BIT);
end component;
component FD port ( C, D: in BIT;Q: out BIT);
end component;
component OBUF port ( I: in BIT;O: out BIT);
end component;
component IBUF port ( I: in BIT;O: out BIT);
end component;
component LUT26 port ( I0,I1: in BIT;O: out BIT);
end component;
signal c3_IBUF_BUFG_0 : BIT;
Since there is no cell BUFG available it is replaced with BUFGP and the O and I lines are sorted correctly.
There is no cell FD available, so a new cell was created.
The result is: SGrayBinary_synthesisElectric.vhd
Then a layout is generated with 'Silicon Compiler' and LTSPICE code is added.
Be sure to select a M1 line in any layout cell to avoid error:
"SC Maker cannot find Horizontal Arc Metal-1 in technology artwork"
In "File - Preferences - Tools - Silicon Compiler" the number of rows can be changed.
Synthesized layout
Add LTSPICE simulation code to layout.
.global VDD
.option TEMP 25
VDD VDD 0 DC 1
VC3 C3 0 PULSE(0 1 0n 1n 1n 4n 320n)
VC2 C2 0 PULSE(0 1 10n 1n 1n 10n 20n)
VGD GD 0 PULSE(0 1 50n 1n 1n 50n 400n)
.include cmosedu_models.txt
.tran 0 2800n
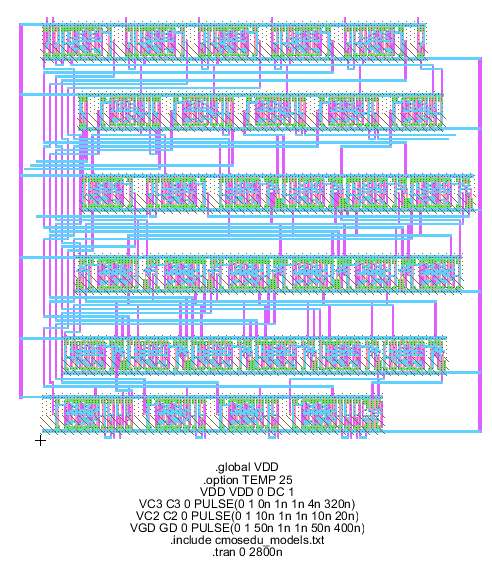
Input vectors for TestJS.html
Xilinx has asynchronous reset with C3. FDC in sclib has synchronous reset.
C2 has to do a clock cycle while C3 active.
Use TestJS to create LTSPICE simulation commands.
Paste the test vectors into the input field and 'Generate analysis request'.
C2,C3,GD;xD15,xD14,xD13,xD12,xD11,xD10,xD9,xD8,xD7,xD6,xD5,xD4,xD3,xD2,xD1,xD0;COMMENT
0, 1, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 1, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; Reset
0, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "1" 15
0, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "1"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0" 11
0, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "1"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0" 7
0, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "1"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0"
0, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 1; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "1" 3
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0"
0, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 0, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0; GD "0"
0, 1, 0; 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0;
1, 1, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; Reset expect 878f
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0" 15
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0"
0, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "1"
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0"
0, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "1" 11
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0"
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0"
0, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "1"
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0" 7
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0"
0, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "1"
0, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "1"
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0" 3
0, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 1; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "1"
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0"
0, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 0, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1; GD "0"
0, 1, 0; 1,0,0,0,0,1,1,1,1,0,0,0,1,1,1,1;
1, 1, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; Reset 31d8
0, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "0" 15
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1"
0, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "0"
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1"
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1" 11
0, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "0"
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1"
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1"
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1" 7
0, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "0"
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1"
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1"
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1" 3
0, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 1; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "1"
0, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "0"
0, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 0, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0; GD "0"
0, 1, 0; 0,0,1,1,0,0,0,1,1,1,0,1,1,0,0,0;
1, 1, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0; Reset 6d28
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;
0, 0, 0; 0,1,1,0,1,1,0,1,0,0,1,0,1,0,0,0;

Paste LTSPICE code for voltage sources as PWL into LTSPICE.
LTSPICE simulation
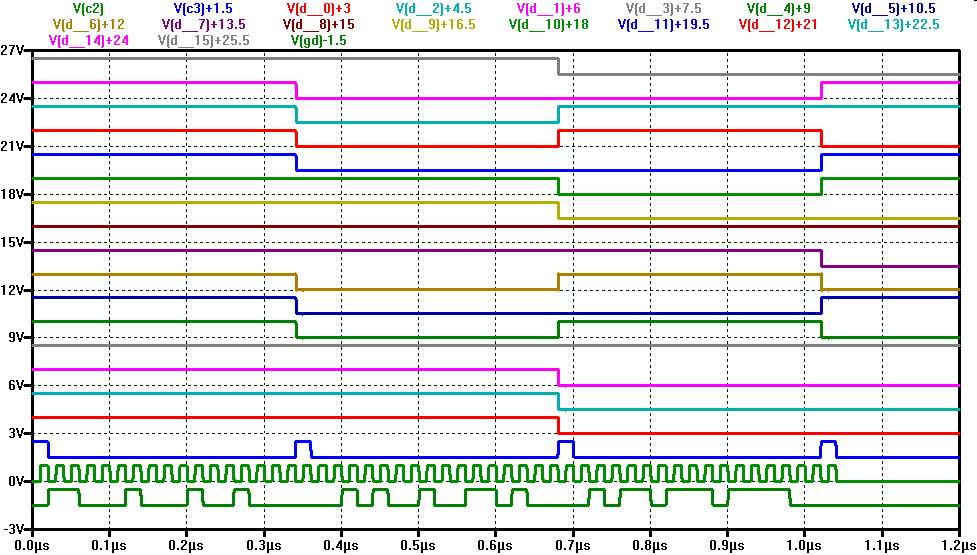
4 short c3 pulses can be seen initializing conversion at 0V level.
Below is c2 clock for acquisition of gd data below.
The correct output data can be seen after 0.35us, 7us and 1.5us.
Create a chip adding Pads (, test structures and alignment marks)
Use and load the library Pads4u.jelib.
Keep SGrayBinary as the "Current Library".
Create a pad frame placing file (.arr) according to Help.
Search in "Help" - "User's Manual" for 'padframe'.
Copy the example padframe in a file. (.arr)
Modify the file according to the inputs and outputs.
Insert in the line core your top level circuit layout name.
Names are case sensitiv.
Try to make a square pad frame:
Text file: sGrayPad.arr
Tools - Generation - Pad Frame Generator
Tools - Routing - Sea of Gates Route
Remaining steps for production
- Chip identifier name is placed
- Alignment marks are placed
- Multiple chips are placed on one reticle
- Test structures are placed on or between the chips
- Fill structures are placed to generate a regular pattern
- Mask generation is started for each layer
- Layers can be resized for correct pattern transfer
LTSPICE code analysis
SUBCKT
Bottom up:
PAD_corner: delete
padpart_bondpad: delete
padpart_pwrrails: delete
PAD_gnd:
Top level:
PAD_corner: dgndL dvddL welltapL delete
PAD_gnd: dgndL dvddL gnd_in
PAD_in:
PAD_out:
PAD_spacer: delete
PAD_vdd: dgndL dvddL m1m2 welltapL
IRSIM
IRSIM is a switch level simulator.
In Electric operation is difficult.
Tools - Simulation(Built-in) - IRSIM: Simulate current cell
Tools - Simulation(Built-in) - Restore Stimuli from disk
Stimuli are loaded from a file GraySim.cmd
Deliverables
Document your laboratory in a pdf document with a name of < Date > _2020_SGray_ < name > .pdf
and send it to joerg.vollrath@hs-kempten.de.
A maximum of 2 students can prepare a document together clearly marking authorship
of different sections. Submission is due 10.7.2019.
- Schematic of switch matrix or LUT
- LTSPICE (and IRSIM) simulation of this block with extensive comments
- < Name > .jelib with Chip_FPGA, FPGA, LUT2 or MUX41 and DEMUX14 and simulation code
- LTSPICE simulation of FPGA.
- Discussion of architecture options.
- Table listing time efforts to complete the laboratory.
- Summary
Result
Reticle